LeetCode는 프로그래밍 문제를 풀며 코딩 실력을 향상할 수 있는 온라인 플랫폼입니다. 다양한 알고리즘 및 데이터 구조 문제를 제공하며, 면접 대비에 유용합니다. 해당 문제는, LeetCode Problems에서 볼 수 있는 난이도 '쉬움 (Easy)' 단계인 "Valid Word" 문제입니다.
--> https://leetcode.com/problems/valid-word/description/
문제 :
A word is considered valid if: It contains a minimum of 3 characters.
It contains only digits (0-9), and English letters (uppercase and lowercase).
It includes at least one vowel. It includes at least one consonant.
You are given a string word.
Return true if word is valid, otherwise, return false.
Notes: 'a', 'e', 'i', 'o', 'u', and their uppercases are vowels.
A consonant is an English letter that is not a vowel.
Example 1 :
Input : word = "234Adas"
Output : true
Explanation : This word satisfies the conditions.
Example 2 :
Input : word = "b3"
Output : false
Explanation : The length of this word is fewer than 3, and does not have a vowel.
Example 3 :
Input : word = "a3$e"
Output : false
Explanation : This word contains a '$' character and does not have a consonant.
Constraints :
- 1 <= word.length <= 20
- word consists of English uppercase and lowercase letters, digits, '@', '#', and '$'.
이 문제는 주어진 문자열이 유효한 단어인지 확인하는 문제입니다. 유효한 단어의 조건은 길이가 최소 3 이상이어야 하고, 숫자와 영문자(대소문자)만 포함해야 하며, 최소 하나의 모음과 자음이 있어야 합니다. 예를 들어, "234Adas"는 조건을 만족하므로 유효한 단어입니다.
class Solution {
public boolean isValid(String word) {
boolean consoExist = false;
boolean vowelExist = false;
int vowCount = 0;
int conCount = 0;
if (word.length() < 3) return false;
for (int i = 0; i < word.length(); i++) {
char c = word.charAt(i);
if (Character.isDigit(c)) {
} else if (Character.isLetter(c)) {
if (isVowel(c)) vowCount++;
else conCount++;
} else {
return false;
}
}
if (vowCount >= 1 && conCount >= 1) {
consoExist = true;
vowelExist = true;
}
return consoExist && vowelExist;
}
private static boolean isVowel(char c) {
c = Character.toLowerCase(c);
return c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u';
}
}
제 코드는 정말 반복문과 조건문으로만 이뤄진 심플한 코드입니다 :
- 초기 조건 확인 :
- 단어 길이가 3보다 작으면 바로 false를 반환합니다.
- 문자 순회 및 유효성 검사 :
- 각 문자를 순회하면서 숫자인지, 문자(모음/자음)인지 확인합니다.
- 숫자나 문자 이외의 문자가 있으면 바로 false를 반환합니다.
- 모음과 자음 존재 여부 확인 :
- 모음과 자음이 모두 하나 이상 있는지 확인합니다.
- 결과 반환 :
- 모음과 자음이 모두 존재하면 true를 반환합니다.
- 모음 확인 함수 :
- 문자가 모음인지 확인하는 함수입니다. 대소문자를 구분하지 않도록 소문자로 변환하여 체크합니다.
시간 및 공간 복잡도 :
- 시간 복잡도 :
- O(n) : 문자열의 각 문자를 한 번씩 순회하므로 시간 복잡도는 O(n)입니다. 여기서 n은 문자열의 길이입니다.
- 공간 복잡도 :
- O(1) : 추가적인 데이터 구조를 사용하지 않으므로 공간 복잡도는 O(1)입니다.
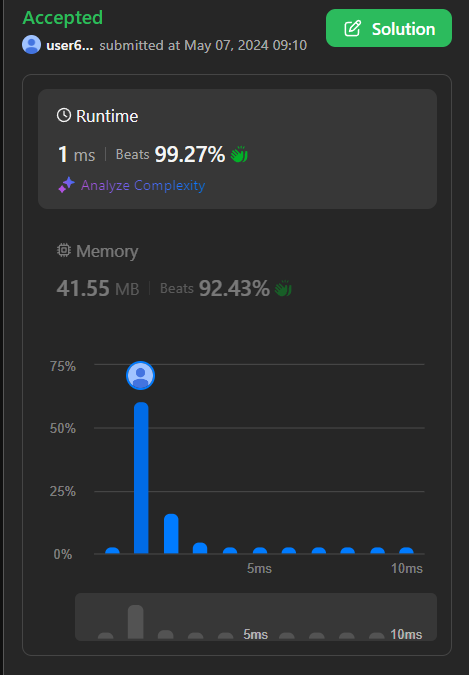
'LeetCode' 카테고리의 다른 글
[LeetCode] - 2894. Divisible and Non-Divisible Sums Difference (0) | 2024.07.26 |
---|---|
[LeetCode] - 3099. Harshad Number (0) | 2024.07.26 |
[LeetCode] - 1137. N-th Tribonacci Number (0) | 2024.07.25 |
[LeetCode] - 70. Climbing Stairs (0) | 2024.07.25 |
[LeetCode] - 1387. Sort Integers by the Power Value (0) | 2024.07.25 |